Java JDBC provides a standard interface to interact with any relational databases. In this tutorial series, you will learn how to use the MySQL JDBC Connector to connect Java programs to MySQL databases.
What you’ll learn
- Overview of JDBC
- Setting up MySQL JDBC environment
- Perform common database operations including selecting, inserting, updating, and deleting data.
- Transactions
- Calling stored procedures
- Working with BLOB data
Prerequisite
- Basic knowledge of MySQL
- Fundamental Java programming
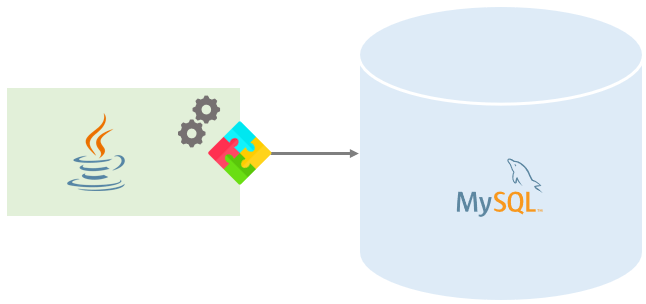
Section 1. Getting Started
- Introduction to JDBC – Learn about JDBC so that you can use it for interacting with MySQL databases.
- Setting Up MySQL JDBC Development Environment – Show you how to set up a development environment that helps you work with MySQL and JDBC.
- Connecting to MySQL Using JDBC Driver – Learn how to connect to the MySQL database using the JDBC Connection object.
Section 2. CRUD
- Querying Data From MySQL Using JDBC – Guide you on how to query data from MySQL using JDBC Statement and ResultSet objects.
- Updating Data in MySQL Using JDBC PreparedStatement -Learn how to update data in the MySQL database using the JDBC PreparedStatement interface.
- Inserting Data Into Table Using JDBC PreparedStatement – Learn how to use the PreparedStatement object to insert data into a table and get the inserted ID back.
Section 3. Transaction
- MySQL JDBC Transaction – Learn how to use commit() and rollback() methods of the Connection object to control transactions.
Section 4. Calling MySQL Stored Procedures
- Calling MySQL Stored Procedures from JDBC – Show you how to call MySQL stored procedures from JDBC using the CallableStatement object.
Section 5. Working with BLOB
- Writing and Reading MySQL BLOB Using JDBC – Show you how to write and read MySQL BLOB data using JDBC API.