Node.js offers several packages to interact with MySQL databases. One of the most popular packages is mysql.
The mysql
package facilitates the execution of SQL queries, allowing you access to MySQL seamlessly.
Whether you are developing API, server-side applications, or web applications, the mysql package simplifies database interactions using Node.js.
In this tutorial series, you will learn how to master the mysql
package from scratch. You will learn how to perform common operations including:
- Connecting MySQL Server
- Creating a new table
- Inserting data into a table
- Querying data from a table
- Updating data
- Deleting data
- Calling a stored procedure
After the tutorial, you will be able to develop the Node.js application using MySQL as the back-end database.
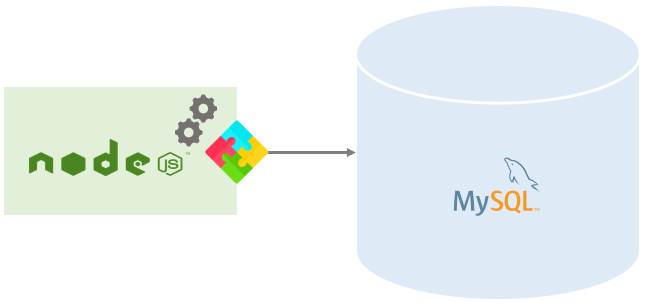
Section 1. Getting Started
- Connecting to the MySQL Database Server from Node.js – Connect to the MySQL database server from a node.js application using the mysql module API.
- Creating Tables in MySQL from Node.js – Create a new table in a MySQL database from a Node.js application using the mysql module.
Section 2. CRUD
- Inserting Rows Into a Table from Node.js – Learn how to insert one or more rows into a table from the Node.js application.
- Querying Data in MySQL Database from Node.js – Query data from a table in the MySQL database from a Node.js application using the mysql module.
- Updating Data in MySQL Database from Node.js – Learn how to update data in the MySQL database from a Node.js application.
- Deleting Data in MySQL from Node.js – Delete data in the MySQL database from a Node.js application.
Section 3. Stored Procedures
- Connecting to the MySQL Database Server from Node.js – Connect to the MySQL database server from a node.js application using the mysql module API.